This tutorial will demonstrate how to use driver.getData function to read device address data.
Project Design
Includes:
- A Numeric object (Address: LW-100, Type: Uint16)
- A JS object that, when clicked, uses driver.getData function to read LW-100/Uint16 and then outputs the result to JS console.
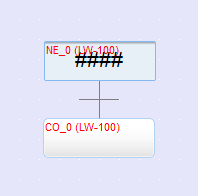
Numeric Object Settings
- In the [General] settings page, set the Read/Write address to LW-100.
- In the [Format] settings page, set the Device data format to 16-bit Unsigned.
JS Object Settings
-
In the [Config] settings page, add an address named "numericAddress", pointing to LW-100, with data type set to 16-bit Unsigned.
- Right-click on config in the table and select [New Value...].
- Expected software display after completing the steps:
-
In the [Shape] settings, select an image from System Button picture library.
Source Code
// Create a MouseArea widget
let ma = new MouseArea();
// Bind the MouseArea to the JS object
this.widget.add(ma);
// When MouseArea triggers the 'mousedown' event, set the current state of the JS object to 1
ma.on("mousedown", (mouseEvent) => {
this.state = 1;
});
// When MouseArea triggers the 'mouseup' event, set the current state of the JS object to 0
ma.on("mouseup", (mouseEvent) => {
this.state = 0;
});
// When MouseArea triggers the 'click' event, read the numericAddress and output the result
ma.on("click", (mouseEvent) => {
driver.getData(this.config.numericAddress, 1, (err, data) => {
if (err) {
console.log('Error:', err.message);
} else {
console.log(data.values);
}
});
});
Execution Result
-
Change the value of LW-100 using the Numeric object.
-
Click the JS object, open cMT Diagnoser, and observe the JS console displaying the updated Numeric value.
Explanation
- driver.getData (function)
- Used to read data from device addresses.
- Response Callback Function (The 3rd parameter)
- Data reading may take time, so a callback function is used to receive the device response without blocking the program.
- After sending a data request, the program will continue execution until either "data is successfully read" or "an error occurs", at which point the result is notified through the callback function.
- The following code replaces driver.getData with driver.promises.getData. This method uses JavaScript's Promise object, which allows for handling asynchronous operations more intuitively. The benefit of using Promise is that the code becomes easier to read and maintain, especially when dealing with multiple asynchronous operations.
ma.on("click", async (mouseEvent) => {
try {
let data = await driver.promises.getData(this.config.numericAddress, 1);
console.log(data.values);
} catch (err) {
console.log('Error:', err.message);
}
});
References
- JS Action/Object SDK
- MDN Web Docs