This tutorial builds on Tutorial 6, aiming to further enhance the capabilities of the "custom object". It will also introduce options that enable users to choose the most suitable settings for their specific context.
Project Design
-
Based on the JS object from Tutorial 6, add "Switch style" property to support the following four modes:
- Set ON
- Set OFF
- Toggle
- Momentary
(This is equivalent to the [Switch style] property of the EB Pro [Toggle Switch] object.)
JS Object Settings
- In the [Config] settings page, add a string named 'switchStyle', with the value set to 'Set ON', 'Set OFF', 'Toggle', or 'Momentary'.
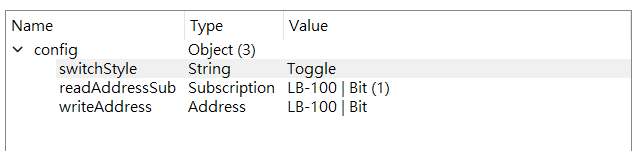
Source Code
// Use Subscription to monitor data changes at the Read address
this.config.readAddressSub.onResponse((err, data) => {
if (err) {
console.log('[response] error =', err.message);
} else {
// Based on the data from the Read address, set the JS object's current state to 0 or 1
this.state = (data.values[0]) ? 1 : 0;
}
});
// Create a MouseArea widget
var ma = new MouseArea();
this.widget.add(ma);
// Based on the configured switch style...
switch (this.config.switchStyle) {
case 'Set ON':
// If the switch style is configured as 'Set ON'
// When the MouseArea emits a 'click' event, write 1 to the Write Address
ma.on("click", (mouseEvent) => {
driver.setData(this.config.writeAddress, 1);
});
break;
case 'Set OFF':
// If the switch style is configured as 'Set OFF'
// When the MouseArea emits a 'click' event, write 0 to the Write Address
ma.on("click", (mouseEvent) => {
driver.setData(this.config.writeAddress, 0);
});
break;
case 'Toggle':
// If the switch style is configured as 'Toggle'
// When the MouseArea emits a 'click' event, if the current state of the JS object is 0, write 1 to the Write Address; otherwise, write 0
ma.on("click", (mouseEvent) => {
driver.setData(this.config.writeAddress, (this.state == 0) ? 1 : 0);
});
break;
case 'Momentary':
// If the switch style is configured as 'Momentary'
// When the MouseArea emits a 'mousedown' event, write 1 to the Write Address
ma.on("mousedown", (mouseEvent) => {
driver.setData(this.config.writeAddress, 1);
});
// When the MouseArea emits a 'mouseup' event, write 0 to the Write Address
ma.on("mouseup", (mouseEvent) => {
driver.setData(this.config.writeAddress, 0);
});
break;
}
Execution Result
- In the [Config] settings page, set the value of 'switchStyle' sequentially to 'Set ON', 'Set OFF', 'Toggle', and 'Momentary'. Execute the simulation and press the JS object to observe whether its behavior aligns with that of the "Toggle Switch" in EB Pro.
Explanation
- The designer and user of a custom object may be different individuals.
- The designer of the custom object should consider the capabilities it will provide and the situations in which it will be applicable during the design phase. If necessary, options should be provided in the [Config] for the user of the object to select appropriate parameters for their specific context.
- When customizing an object using a JS object, the following principles should be adhered to:
- The [Config] should reflect the "parameters" that this custom object is intended to allow the user to configure at the design time of the project, which refers to the object's "properties".
- The [Source code] should reflect the behavior of this custom object during the runtime of the project.