This tutorial will demonstrate how to use driver.setData function to write data to a device address.
Project Design
Includes:
- Two Numeric objects (referred to as A and B) that monitor different device addresses.
- A JS object that writes the data from Numeric A to Numeric B's address whenever there is a change in Numeric A's address data (simulating a data transfer behavior).
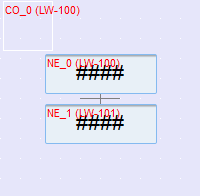
Numeric Object Settings
- Set the Read/Write address of Numeric A to LW-100 and the Device data format to 16-bit Unsigned.
- Set the Read/Write address of Numeric B to LW-101 and the Device data format to 16-bit Unsigned.
JS Object Settings
- In the [Config] settings page, add a subscription named "sourAddress", pointing to LW-100, with the data type set to 16-bit Unsigned and a length of 1.
- Add another address named "destAddress", pointing to LW-101, with the data type set to 16-bit Unsigned.

Source Code
// Use Subscription to monitor data changes at Numeric A's address
this.config.sourAddress.onResponse((err, data) => {
if (err) {
console.log('Error:', err.message);
} else {
// Use driver.setData to write data to Numeric B's address
driver.setData(this.config.destAddress, data.values[0], (err, data) => {
if (err) {
console.log('driver.setData failed, error:', err.message);
} else {
console.log('driver.setData succeeded');
}
});
}
});
Execution Result
-
Change the value of LW-100 using the Numeric object A.
-
Observe that the value of LW-101 within Numeric object B is also updated accordingly.
Explanation
- driver.setData (function)
- Used to write data to a specified device address.
- Response Callback Function (The 3rd parameter)
- Writing data may take time, so a callback function is used to receive the device response without blocking the program.
- After sending a data request, the program continues executing subsequent code until the system notifies of "successful data writing" or "an error occurs" through the callback function.
- If you don't need to know the outcome of driver.setData, this parameter can be omitted.
- The code below replaces driver.setData with driver.promises.setData. This method uses JavaScript's Promise object, which allows for handling asynchronous operations more intuitively. The benefit of using Promise is that the code becomes easier to read and maintain, especially when dealing with multiple asynchronous operations.
this.config.sourAddress.onResponse(async (err, data) => {
if (err) {
console.log('Error:', err.message);
} else {
try {
await driver.promises.setData(this.config.destAddress, data.values[0]);
} catch (error) {
console.log('Error:', error.message);
}
}
});
References
- JS Action/Object SDK